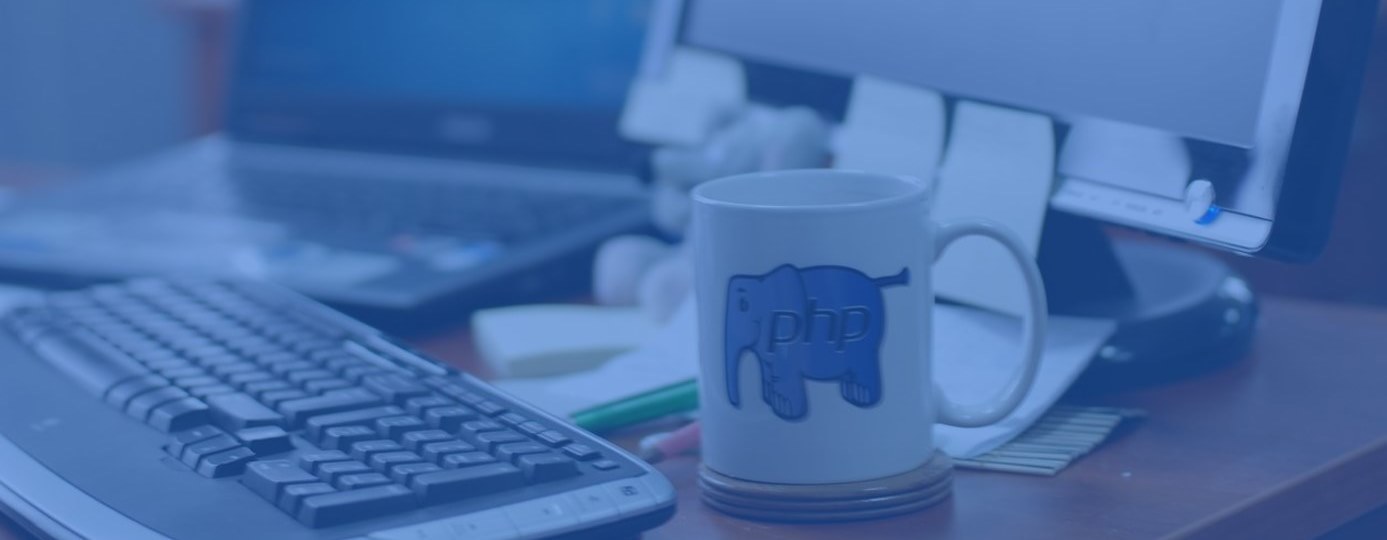
Exploring the GET API Method in PHP
Introduction :
In the world of web development, APIs (Application Programming Interfaces) act as bridges that allow different software applications to communicate and exchange data seamlessly.
Among the variety of HTTP methods available, the POST method holds a crucial role in submitting data to a server. In this tutorial, we will delve into the process of implementing POST API methods in PHP.
By the end of this guide, you’ll be equipped to construct robust APIs capable of handling data submissions like a pro.
Prerequisites :
Before we dive in, let’s make sure you have the necessary groundwork laid out:
Basic understanding of PHP and HTTP concepts.
A local development environment such as XAMPP, WAMP, or MAMP.
A code editor of your choice like Visual Studio Code, PhpStorm, etc.
Table of Contents
1. Understanding the POST Method
The POST method is one of the HTTP methods used for sending data to a server. Unlike GET, which appends data to the URL, POST sends data in the body of the request. This makes it suitable for sensitive or larger amounts of data.
You might use POST for actions like submitting forms, creating new records, or updating existing ones.
2. Setting Up Your PHP Environment
To get started, ensure PHP is installed on your system. You can verify this by running php -v in your terminal. Next, choose a development server (like XAMPP or WAMP) to create a local environment for testing your API.
3. Creating Your First POST API
Begin by setting up a project directory for your API. Inside this directory, create an endpoint to handle POST requests. In PHP, you can use the $_POST superglobal to access POST data. For instance:
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
// Handle POST request
$data = $_POST['data'];
// Process the data further
}
4. Processing POST Data
Retrieve data from the POST request using $_POST, and ensure to sanitize and validate it to prevent security vulnerabilities.
If you intend to store this data in a database, establish a connection to your database (e.g., MySQL) and use prepared statements to prevent SQL injection.
5. Crafting API Responses
After processing the data, construct appropriate HTTP responses using status codes like 200 (OK) or 400 (Bad Request) to indicate success or failure. For response data, using JSON is a popular choice due to its simplicity and compatibility.
header("Content-Type: application/json");
if ($success) {
echo json_encode(["message" => "Data successfully processed"]);
} else {
echo json_encode(["error" => "Data processing failed"]);
}
6. Ensuring API Security :
API security is paramount. Implement measures to prevent SQL injection by using parameterized queries.
Apply input validation to reject malicious data, and guard against Cross-Site Scripting (XSS) attacks by escaping user-generated content.
7. Best Practices for POST APIs
Adhere to RESTful principles, ensuring your API endpoints are named meaningfully. Keep your codebase organized with consistent naming conventions. Document your API thoroughly, providing clear instructions for users.
8. Advanced Topics to Explore
For a more in-depth understanding, explore topics like handling file uploads through POST requests, implementing authentication mechanisms using API keys, and setting up rate limiting to control API usage.
9. Putting It All Together
Congratulations! You’ve now mastered the art of creating and handling POST API methods in PHP. You’ve learned to build secure, efficient, and user-friendly APIs capable of handling data submissions with finesse.
Conclusion :
In this comprehensive guide, we embarked on a journey through the realm of POST API methods in PHP. We explored the fundamental concepts, setup procedures, implementation steps, and best practices for building robust APIs that handle data submissions effectively.
By now, you’ve gained the skills necessary to construct APIs that not only communicate seamlessly but also prioritize security and user experience.
Remember that the POST method plays a pivotal role in transmitting data securely to a server, making it an essential tool for creating and updating resources in your applications. With the knowledge you’ve acquired, you’re well-equipped to navigate the intricacies of handling POST requests, processing data, and crafting meaningful responses.
As you move forward in your development endeavors, always keep in mind the importance of security. Preventing SQL injection, validating input, and safeguarding against XSS attacks are not just best practices but essential components of building trustworthy APIs.