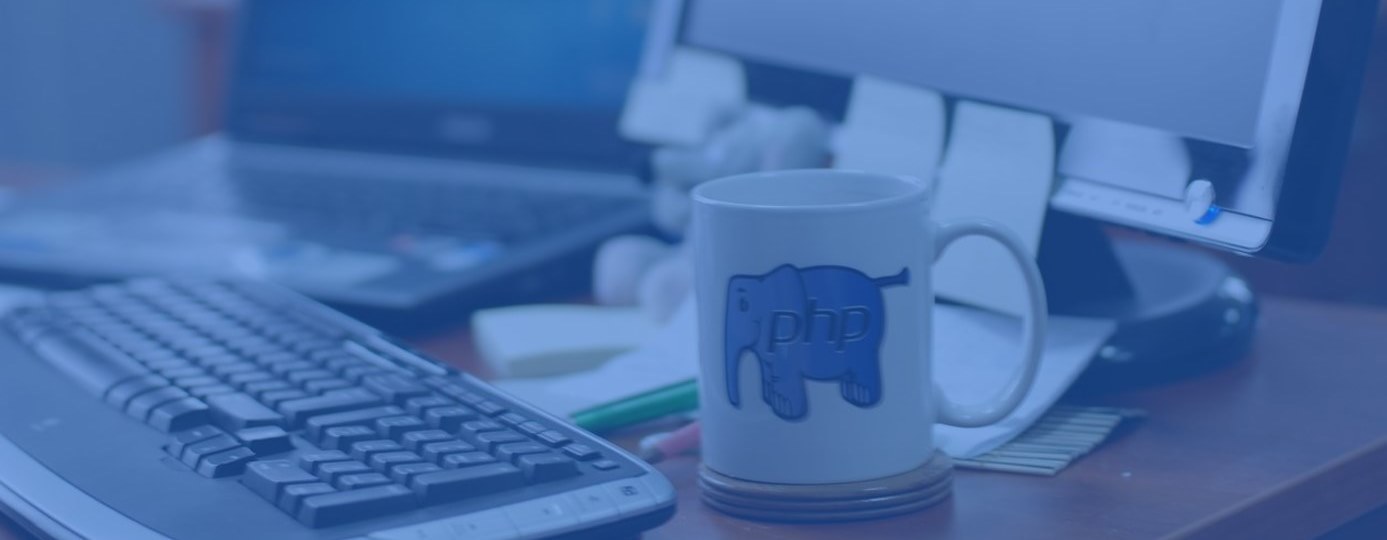
Mastering the DELETE API Method in PHP
Introduction:
- In the realm of web development, data management is a critical aspect of building robust and efficient applications.
- The HTTP DELETE method serves as a fundamental tool for removing resources from a server. In this blog post, we’ll delve into the DELETE API method in PHP, exploring its significance, implementation, and best practices for handling resource deletion.
What is DELETE Api method in PHP?
- The DELETE method sends a request to the server for deleting the request mentioned in the endpoint. Thus, it is capable of updating data on the server.
- Before creating a DELETE request, we shall first send a GET request to the server on the endpoint
Unveiling the DELETE API Method:
- The DELETE method is a standard HTTP method used for requesting the removal of a resource from a server. It’s particularly useful when you want to permanently delete a resource, such as a user profile, a post, or any other data stored on the server.
- By employing the DELETE method, you can effectively manage your application’s data lifecycle.
The Purpose of DELETE:
- The DELETE method is used to instruct the server to remove a specific resource. Upon receiving a DELETE request, the server should take the appropriate action to delete the resource and respond with a relevant status code.
Implementing the DELETE Method in PHP:
- To implement the DELETE API method in PHP, you’ll typically follow these steps:
1. Receive the Request:
- Retrieve the resource identifier from the URL or request parameters.
2. Verify Authorization:
- Ensure that the requester has the necessary permissions to delete the resource.
3. Perform Deletion:
- Delete the resource from your data store, whether it’s a database, file system, or other storage mechanism.
4. Respond to the Request:
- Send an appropriate response status code, indicating the success or failure of the deletion process.
Example Implementation:
if ($_SERVER['REQUEST_METHOD'] === 'DELETE') {
$resourceId = $_GET['id']; // Assuming the resource ID is passed as a query parameter
if (isValidUserToDeleteResource($resourceId)) {
if (deleteResourceFromDatabase($resourceId)) {
http_response_code(204); // 204 No Content
} else {
http_response_code(500); // 500 Internal Server Error
}
} else {
http_response_code(403); // 403 Forbidden
}
} else {
http_response_code(405); // 405 Method Not Allowed
header("Allow: DELETE");
echo "DELETE method not allowed.";
}
Best Practices for DELETE Method:
- Here are some best practices to consider when implementing the DELETE method in PHP:
1. Authentication and Authorization: Ensure that only authorized users can delete resources. Verify user permissions before performing deletions.
2. Idempotence: DELETE requests should be idempotent, meaning that multiple identical requests should have the same effect as a single request.
Deleting a resource multiple times should not lead to unintended consequences.
3. Resource Not Found: If a DELETE request is made for a resource that doesn’t exist, respond with a 404 Not Found status code.
4. Error Handling: If the deletion process encounters errors, provide clear error messages in the response body and use appropriate error status codes.
Conclusion:
- The DELETE API method in PHP plays a pivotal role in managing the lifecycle of resources within your web applications. By implementing this method effectively, you can ensure secure and efficient removal of data from your server.
- Whether you’re building a content management system, an e-commerce platform, or any other web application, mastering the DELETE method empowers you to maintain your data and provide a seamless user experience. Embrace the power of DELETE to keep your applications lean and organized. Happy coding!