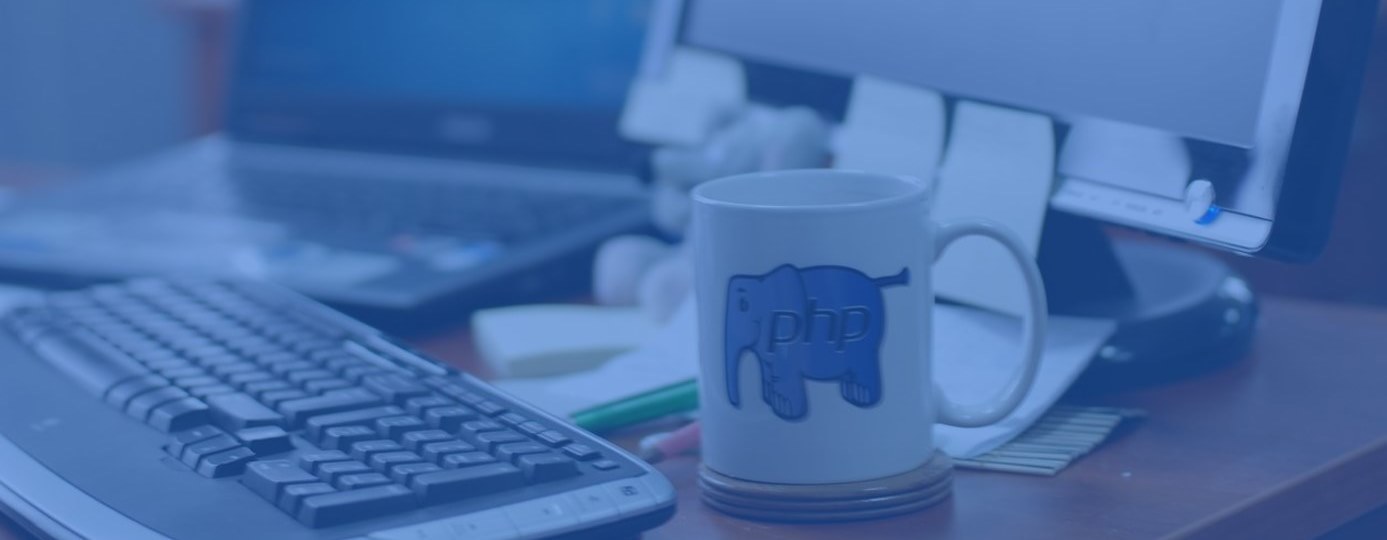
Tracing API Requests in PHP
Introduction :
- In the realm of HTTP methods, the TRACE method often remains overlooked. However, this method holds unique capabilities that can prove invaluable in understanding the journey of an API request through the intricate web of servers and proxies.
- In this blog post, we’ll delve into the TRACE API method in PHP, exploring its purpose, mechanics, and potential applications for debugging and diagnostics.
What is TRACE Api Method in PHP
- A trace tracks an incoming request to your API and the various events (such as RPC calls or instrumented sections of code), along with precise timings of each event.
- These events are represented as spans in the trace.
Unveiling the TRACE API Method:
- The TRACE method is an HTTP method designed for diagnostics and debugging. It allows you to trace the path of a request as it passes through various intermediaries between the client and the server.
- When a TRACE request is made, the server echoes back the received request message, showing the headers as they were received.
- This functionality enables developers to identify misconfigurations, proxy issues, and other anomalies in the request’s journey
The Purpose of TRACE:
The TRACE method serves two primary purposes:
1. Diagnostics: Developers can use the TRACE method to observe how a request changes as it traverses different network components, such as proxies and load balancers.
2. Security: The TRACE method can help identify potential security vulnerabilities related to Cross-Site Tracing (XST) attacks, allowing developers to implement necessary safeguards.
Using the TRACE Method in PHP:
- To demonstrate the TRACE method in PHP, let’s create a simple example:
if ($_SERVER['REQUEST_METHOD'] === 'TRACE') {
header("Content-Type: message/http");
echo $_SERVER['REQUEST_METHOD'] . " " . $_SERVER['REQUEST_URI'] . " " . $_SERVER['SERVER_PROTOCOL'] . "\r\n";
foreach ($_SERVER as $key => $value) {
if (strpos($key, 'HTTP_') === 0) {
echo substr($key, 5) . ": " . $value . "\r\n";
}
}
} else {
http_response_code(405);
header("Allow: TRACE");
echo "TRACE method not allowed.";
}
In this example, if a TRACE request is made to the PHP script, it responds by echoing back the received request headers in the format of an HTTP message.
Benefits of Using the TRACE Method:
The TRACE method offers several benefits for PHP developers and system administrators:
1. Request Analysis: TRACE helps developers visualize the modifications made to a request as it flows through the network, facilitating debugging and troubleshooting.
2. Proxy Debugging: TRACE is particularly useful for identifying misconfigurations or modifications introduced by intermediate proxies, load balancers, or firewalls.
3. Security Insights: The TRACE method can help uncover potential vulnerabilities related to Cross-Site Tracing attacks, allowing you to take proactive security measures.
4. Diagnostics: When troubleshooting complex systems, TRACE provides a real-time view of the request’s journey, aiding in diagnosing issues related to headers, encoding, or other request attributes.
Conclusion:
- The TRACE API method might not be a daily tool in every PHP developer’s toolbox, but its unique capabilities can be invaluable in diagnosing complex issues and understanding how requests navigate through the intricacies of the web.
- By leveraging the TRACE method, you can gain insights into the behavior of your API requests, identify anomalies, and improve both the security and performance of your applications. So, when the need arises, remember that TRACE is there to shed light on the path your requests take. Happy tracing!